Emissive Shape Creation
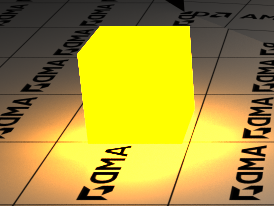
Emissive shapes are simply rpr_shapes created with rprContextCreateMesh() with an RPR_MATERIAL_NODE_EMISSIVE material.
We need to create both a shape and an emissive material. Note that the amount of light emitted (radiance) is per unit area, so a larger light will emit more light. Often this behavior is undesired, in which case the ‘color’ parameter to RPR_MATERIAL_NODE_EMISSIVE should be scaled down with the surface area of the geometry.
// Create a light
rpr_shape light;
{
CHECK( rprContextCreateMesh(context,
(rpr_float const*)&plane_data[0], 4, sizeof(vertex),
(rpr_float const*)((char*)&plane_data[0] + sizeof(rpr_float)*3), 4, sizeof(vertex),
(rpr_float const*)((char*)&plane_data[0] + sizeof(rpr_float)*6), 4, sizeof(vertex),
(rpr_int const*)indices, sizeof(rpr_int),
(rpr_int const*)indices, sizeof(rpr_int),
(rpr_int const*)indices, sizeof(rpr_int),
num_face_vertices, 2, &light) );
// Create a transform
RadeonProRender::matrix lightm = RadeonProRender::translation(RadeonProRender::float3(0,8,2));
// Set the transform for the light
CHECK( rprShapeSetTransform(light, RPR_TRUE, &lightm.m00) );
// Create an emissive material
rpr_material_node emissive;
{
CHECK( rprMaterialSystemCreateNode(matsys, RPR_MATERIAL_NODE_EMISSIVE, &emissive) );
// Set diffuse color parameter to red
CHECK( rprMaterialNodeSetInputF(emissive, "color", 3.5f, 0.0f, 0.0f, 0.f) );
// Apply the material on the shape
CHECK( rprShapeSetMaterial(light, emissive) );
}
// Attach the light to the scene
CHECK( rprSceneAttachShape(scene, light) );
}
An example of creating an emissive shape can be found in the Basic Scene demo.