Materials Demo
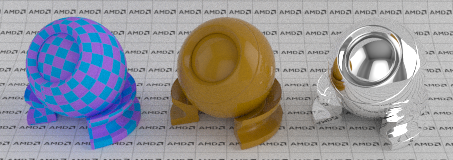
The material tutorial illustrates how to create materials in RPR. Note that it does not cover Uber materials (RPR_MATERIAL_NODE_UBERV2). The case of Uber materials is shown in detail in the Uber Material tutorial.
In order to have a source code focusing on the material, we are using a MatballScene
class (defined inside common.cpp and using the concepts introduced inside the Basic Scene demo) to manage all the scene setup, filled with Material Ball or ‘matball’ models.
This tutorial currently illustrates three material graphs (more materials may be added in the future).
The matball on the left illustrates the procedural texture: here we use a Checker as the weighting coefficient for a Blend between two Diffuse colors.
The matball in the middle is a Blend between a Diffuse material and a Microfacet material, using a Fresnel as the weighting coefficient.
The matball on the right is a simple Reflection material.
Example of a source code to create a layered material: blending a Diffuse and a Microface (matball in the middle).
// Create the first layer
rpr_material_node base = nullptr;
CHECK(rprMaterialSystemCreateNode(matballScene.m_matsys, RPR_MATERIAL_NODE_DIFFUSE, &base));
CHECK(rprMaterialNodeSetInputFByKey(base, RPR_MATERIAL_INPUT_COLOR, 0.5f, 0.25f, 0.f, 1.f)); // Diffuse color
// Create the second layer
rpr_material_node top = nullptr;
CHECK(rprMaterialSystemCreateNode(matballScene.m_matsys, RPR_MATERIAL_NODE_MICROFACET, &top));
CHECK(rprMaterialNodeSetInputFByKey(top, RPR_MATERIAL_INPUT_COLOR, 1.f, 1.f, 1.f, 1.f)); // Specular color
CHECK(rprMaterialNodeSetInputFByKey(top, RPR_MATERIAL_INPUT_ROUGHNESS, 0.05f, 0.f, 0.f, 1.f)); // Roughness
// Material used to weigh the two layers
rpr_material_node fresnel = nullptr;
CHECK(rprMaterialSystemCreateNode(matballScene.m_matsys, RPR_MATERIAL_NODE_FRESNEL, &fresnel));
CHECK(rprMaterialNodeSetInputFByKey(fresnel, RPR_MATERIAL_INPUT_IOR, 1.4f,0.0f,0.0f,0.0f ));
// Create the blender material
rpr_material_node layered = nullptr;
CHECK(rprMaterialSystemCreateNode(matballScene.m_matsys, RPR_MATERIAL_NODE_BLEND, &layered));
CHECK(rprMaterialNodeSetInputNByKey(layered, RPR_MATERIAL_INPUT_COLOR0, base)); // Set material for base layer
CHECK(rprMaterialNodeSetInputNByKey(layered, RPR_MATERIAL_INPUT_COLOR1, top)); // Set material for top layer
CHECK(rprMaterialNodeSetInputNByKey(layered, RPR_MATERIAL_INPUT_WEIGHT, fresnel));
CHECK(rprShapeSetMaterial(plane, layered));