Textured Material Creation
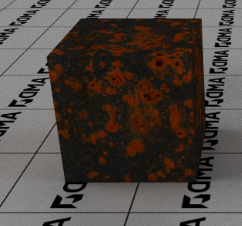
There are numerous material nodes that can be used. Nodes are atomic pieces of materials which calculate an output color based on inputs. Inputs can be floating point values, textures or even other nodes. By connecting nodes together with inputs, a ‘node tree’ can be formed which calculates a complex material.
In order to input an image inside a material graph, we need to use the RPR_MATERIAL_NODE_IMAGE_TEXTURE material. This node takes an rpr_image as input and outputs the sampled colors.
rpr_material_node diffuse1 = nullptr;
rpr_image img1 = nullptr;
rpr_material_node tex = nullptr;
{
// First, create the rpr_image
const std::string pathImageFileA = "../../Resources/Textures/concrete_worn_bare_Base_Color.jpg";
status = rprContextCreateImageFromFile(context, pathImageFileA.c_str(), &img1);
if (status == RPR_ERROR_IO_ERROR)
{
std::cout << "Error : " << pathImageFileA << " not found.\n";
return -1;
}
CHECK(status);
// Create the IMAGE_TEXTURE material, used to sample the image data
CHECK(rprMaterialSystemCreateNode(matsys, RPR_MATERIAL_NODE_IMAGE_TEXTURE, &tex));
// Set image data to the material
CHECK(rprMaterialNodeSetInputImageDataByKey(tex, RPR_MATERIAL_INPUT_DATA, img1));
// Create a simple diffuse material to render the image
CHECK(rprMaterialSystemCreateNode(matsys, RPR_MATERIAL_NODE_DIFFUSE, &diffuse1));
// The color from the image will be used as input of the diffuse material
CHECK(rprMaterialNodeSetInputNByKey(diffuse1, RPR_MATERIAL_INPUT_COLOR, tex));
// Attach the diffuse material to the shape
CHECK(rprShapeSetMaterial(plane, diffuse1));
}
Examples of creating textured materials can be found in the Basic Scene demo.